This article will help you to show how to add custom pattern and validation error message for a text field in the admin panel.
First, you need to create a category attribute using a module setup script and you can add validation on the XML file.
Add the setup script to create a category attribute in the following file.
app/code/MilanDev/CustomField/Setup/InstallData.php
<?php
namespace MilanDev\CustomField\Setup;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
/**
* Constructor
*
* @param \Magento\Eav\Setup\EavSetupFactory $eavSetupFactory
*/
public function __construct(EavSetupFactory $eavSetupFactory)
{
$this->eavSetupFactory = $eavSetupFactory;
}
/**
* {@inheritdoc}
*/
public function install(
ModuleDataSetupInterface $setup,
ModuleContextInterface $context
) {
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->addAttribute(
\Magento\Catalog\Model\Category::ENTITY,
'my_custom_field',
[
'type' => 'varchar',
'label' => 'My Custom Field',
'input' => 'text',
'sort_order' => 333,
'source' => '',
'global' => 1,
'visible' => true,
'required' => false,
'user_defined' => false,
'default' => null,
'group' => 'General Information',
'backend' => ''
]
);
}
}
Now, add the custom pattern and validation error message in the following.
app/code/MilanDev/CustomField/view/adminhtml/ui_component/category_form.xml
<?xml version="1.0" ?>
<form xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Ui:etc/ui_configuration.xsd">
<fieldset name="general">
<field name="my_custom_field">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="required" xsi:type="boolean">false</item>
<item name="sortOrder" xsi:type="number">333</item>
<item name="dataType" xsi:type="string">string</item>
<item name="formElement" xsi:type="string">input</item>
<item name="label" translate="true" xsi:type="string">My Custom Field</item>
<item name="validation" xsi:type="array">
<item name="required-entry" xsi:type="array">
<item name="validate" xsi:type="boolean">true</item>
<item name="message" xsi:type="string">Custom error message for required field.</item>
</item>
<item name="pattern" xsi:type="array">
<item name="validate" xsi:type="boolean">true</item>
<item name="value" xsi:type="string">/^[A-Za-z]+[A-Za-z0-9_]+$/i</item>
<item name="message" xsi:type="string">Custom error message for pattern validation</item>
</item>
</item>
</item>
</argument>
</field>
</fieldset>
</form>
After following the above steps you can input the wrong characters and check. You should see something in the screenshot.
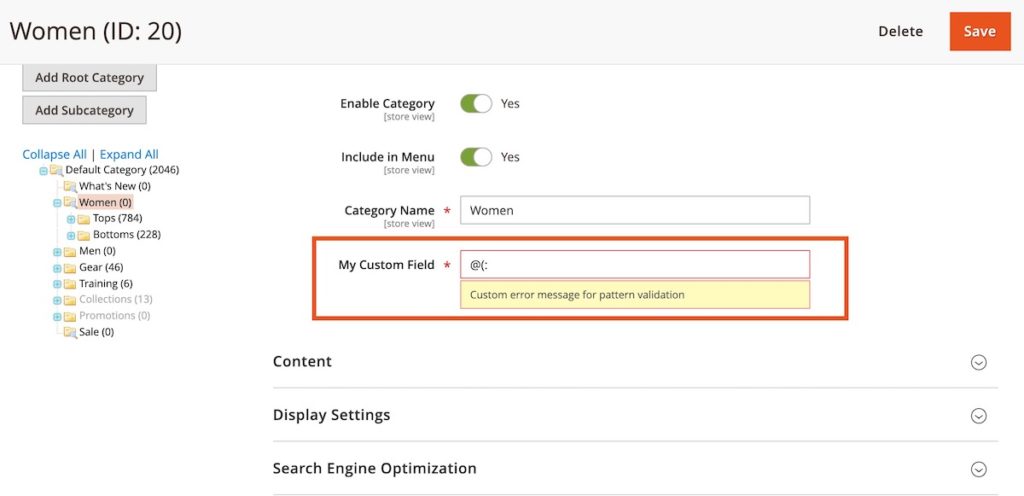
I hope, these tips and tricks will help you. Happy Coding.